
How To Enclose Vstack Inside Rectangle
January 23, 2025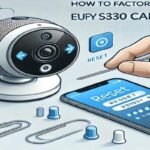
How To Factory Reset Eufy S3330 Cam
January 23, 2025In Java, ending a thread midway requires careful handling to ensure proper resource management and avoid potential issues. Here’s a guide to achieve this safely and effectively.
Why End a Thread Midway?
- Improve Performance: Stop unnecessary threads to optimize application performance.
- Prevent Deadlocks: Avoid system hang-ups by terminating problematic threads.
- Enhance Control: Manage application flow more precisely by stopping threads as needed.
Steps to End a Thread Midway in Java
- Use a Flag Variable:
Implement a volatile boolean variable to signal thread termination.
public class MyThread extends Thread {
private volatile boolean running = true;
public void run() {
while (running) {
// Thread logic here
}
}
public void stopRunning() {
running = false;
}
}
- Call the Stop Method:
Invoke the custom stopRunning() method from the main thread to terminate it.
MyThread thread = new MyThread();
thread.start();
// Perform operations
thread.stopRunning();
- Handle InterruptedException:
For threads using sleep() or wait(), handle InterruptedException to stop gracefully.
public void run() {
while (running) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
break;
}
}
}
- Avoid Using Thread.stop():
- Avoid the deprecated Thread.stop() method as it can cause resource leaks and corruption.
- Use ExecutorService for Thread Pools:
For better control, manage threads using ExecutorService and its shutdownNow() method.
ExecutorService executor = Executors.newSingleThreadExecutor();
executor.submit(() -> {
while (!Thread.currentThread().isInterrupted()) {
// Task logic
}
});
executor.shutdownNow();
Tips for Better Thread Management
- Minimize Critical Sections: Keep critical sections short to reduce thread interference.
- Log Actions: Log thread termination events for better debugging and monitoring.
- Use Thread-safe Operations: Ensure shared resources are properly synchronized.
Troubleshooting Common Issues
- Thread Does Not Stop:
- Check if the flag variable is updated and accessible to the thread.
- InterruptedException Not Handled:
- Ensure all sleep() or wait() calls handle interruptions correctly.
- Resource Leaks:
- Release resources such as file handles or database connections before stopping threads.
Also Read: How To Enclose Vstack Inside Rectangle
Conclusion
Ending a thread midway in Java is a powerful tool for effective application control. By following these steps, you can safely and efficiently manage threads while maintaining system stability.